Expo 地图
一个提供对 Android 上的 Google 地图和 iOS 上的 Apple 地图的访问的库。
此库目前处于 alpha 测试阶段,可能会经常发生重大更改。Expo Go 应用中暂不支持此功能 - 请使用 开发构建 进行试用。
安装
¥Installation
-
npx expo install expo-maps
If you are installing this in an existing React Native app, make sure to install expo
in your project.
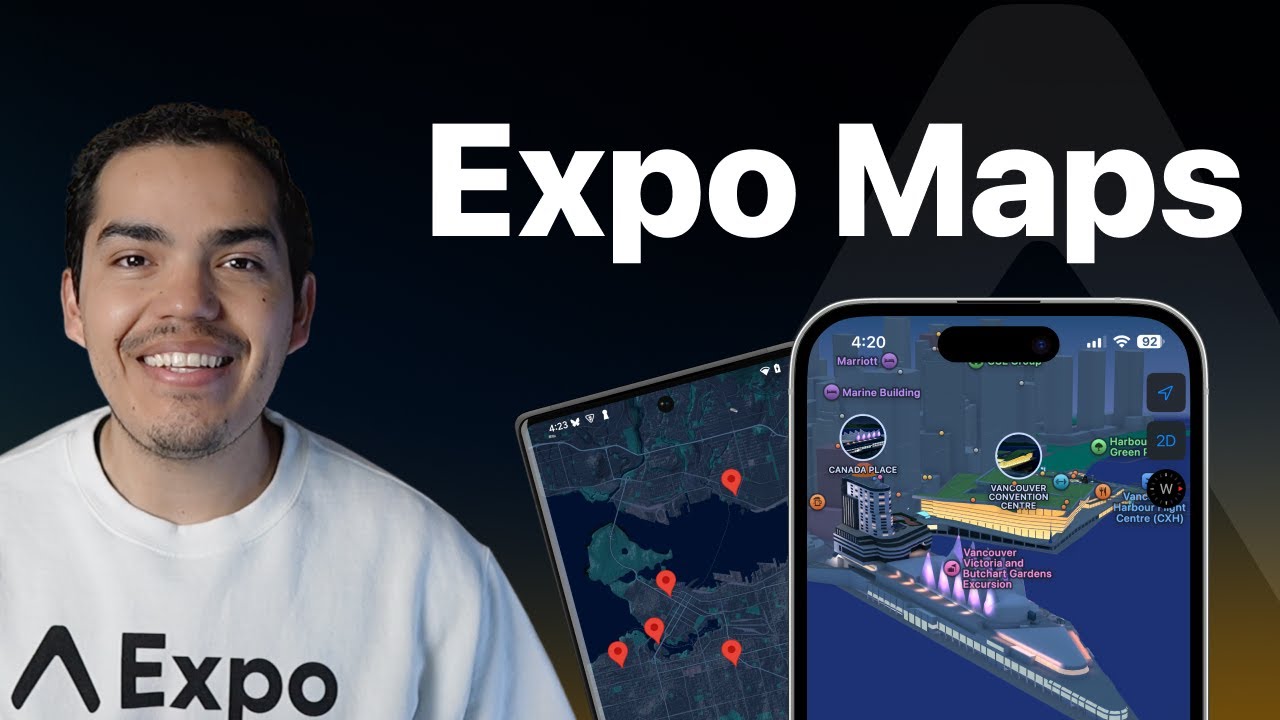
配置
¥Configuration
Expo Maps 提供对 Android 和 iOS 上平台原生地图 API 的访问。
¥Expo Maps provides access to the platform native map APIs on Android and iOS.
-
Apple Maps(仅适用于
iOS)。安装此软件包后,无需额外配置即可使用它。¥Apple Maps (available on
iOSonly). No additional configuration is required to use it after installing this package. -
Google 地图(仅在
Android上可用)。虽然 Google 提供了适用于 iOS 的 Google Maps SDK,但 Expo Maps 仅在 Android 上支持它。如果你想在 iOS 上使用 Google 地图,你可以考虑使用 替代库 或 编写你自己的。¥Google Maps (available on
Androidonly). While Google provides a Google Maps SDK for iOS, Expo Maps supports it exclusively on Android. If you want to use Google Maps on iOS, you can look into using an alternative library or writing your own.
Google Cloud API 设置
¥Google Cloud API setup
在你可以在 Android 上使用 Google 地图之前,你需要注册一个 Google Cloud API 项目,启用 Android 版 Maps SDK,并将相关配置添加到你的 Expo 项目。
¥Before you can use Google Maps on Android, you need to register a Google Cloud API project, enable the Maps SDK for Android, and add the associated configuration to your Expo project.
Set up Google Maps on Android
If you have already registered a project for another Google service on Android, such as Google Sign In, you enable the Maps SDK for Android on your project and jump to step 4.
1
Register a Google Cloud API project and enable the Maps SDK for Android
- Open your browser to the Google API Manager and create a project.
- Once it's created, go to the project and enable the Maps SDK for Android.
2
Copy your app's SHA-1 certificate fingerprint
!!!IG3!!!
!!!IG4!!!
- If you are deploying your app to the Google Play Store, you'll need to upload your app binary to Google Play console at least once. This is required for Google to generate your app signing credentials.
- Go to the Google Play Console > (your app) > Release > Setup > App integrity > App Signing.
- Copy the value of SHA-1 certificate fingerprint.
!!!IG5!!!
!!!IG6!!!
- If you have already created a development build, your project will be signed using a debug keystore.
- After the build is complete, go to your project's dashboard, then, under Configure > click Credentials.
- Under Application Identifiers, click your project's package name and under Android Keystore copy the value of SHA-1 Certificate Fingerprint.
!!!IG7!!!
!!!IG8!!!
3
Create an API key
- Go to Google Cloud Credential manager and click Create Credentials, then API Key.
- In the modal, click Edit API key.
- Under Key restrictions > Application restrictions, choose Android apps.
- Under Restrict usage to your Android apps, click Add an item.
- Add your
android.package
from app.json (for example:com.company.myapp
) to the package name field. - Then, add the SHA-1 certificate fingerprint's value from step 2.
- Click Done and then click Save.
4
Add the API key to your project
- Copy your API Key into your app.json under the
android.config.googleMaps.apiKey
field. - Create a new development build, and you can now use the Google Maps API on Android with
expo-maps
.
Permissions
To display the user's location on the map, you need to declare and request location permission beforehand. You can configure this using the built-in config plugin if you use config plugins in your project (EAS Build or npx expo run:[android|ios]
). The plugin allows you to configure various properties that cannot be set at runtime and require building a new app binary to take effect.
Example app.json with config plugin
!!!IG0!!!
Configurable properties
Name | Default | Description |
---|---|---|
requestLocationPermission | false | A boolean to add permissions to AndroidManifest.xml and Info.plist. |
locationPermission | "Allow $(PRODUCT_NAME) to use your location" | Only for: iOS A string to set the |
Usage
!!!IG1!!!
API
!!!IG2!!!
Components
Type: React.Element<Omit<AppleMapsViewProps, 'ref'>>
(event: {
bearing: number,
coordinates: Coordinates,
tilt: number,
zoom: number
}) => void
Lambda invoked when the map was moved by the user.
(event: {
coordinates: Coordinates
}) => void
Lambda invoked when the user clicks on the map. It won't be invoked if the user clicks on POI or a marker.
(event: AppleMapsMarker) => void
Lambda invoked when the marker is clicked
(event: AppleMapsPolyline) => void
Lambda invoked when the polyline is clicked
Ref<AppleMapsViewType>
StyleProp<ViewStyle>
AppleMapsUISettings
The MapUiSettings
to be used for UI-specific settings on the map.
Type: React.Element<Omit<GoogleMapsViewProps, 'ref'>>
(event: {
bearing: number,
coordinates: Coordinates,
tilt: number,
zoom: number
}) => void
Lambda invoked when the map was moved by the user.
(event: {
coordinates: Coordinates
}) => void
Lambda invoked when the user clicks on the map. It won't be invoked if the user clicks on POI or a marker.
(event: {
coordinates: Coordinates
}) => void
Lambda invoked when the user long presses on the map.
(event: GoogleMapsMarker) => void
Lambda invoked when the marker is clicked
(event: {
coordinates: Coordinates,
name: string
}) => void
Lambda invoked when a POI is clicked.
(event: GoogleMapsPolyline) => void
Lambda invoked when the polyline is clicked.
Ref<GoogleMapsViewType>
StyleProp<ViewStyle>
GoogleMapsUISettings
The MapUiSettings
to be used for UI-specific settings on the map.
GoogleMapsUserLocation
User location, overrides default behavior.
Type: React.Element<GoogleStreetViewProps>
StreetViewCameraPosition
StyleProp<ViewStyle>
Hooks
Parameter | Type |
---|---|
options(optional) | PermissionHookOptions<object> |
Check or request permissions to access the location.
This uses both requestPermissionsAsync
and getPermissionsAsync
to interact with the permissions.
[null | PermissionResponse, RequestPermissionMethod<PermissionResponse>, GetPermissionMethod<PermissionResponse>]
Example
const [status, requestPermission] = useLocationPermissions();
Methods
Checks user's permissions for accessing location.
Promise<PermissionResponse>
A promise that fulfills with an object of type PermissionResponse
.
Asks the user to grant permissions for location.
Promise<PermissionResponse>
A promise that fulfills with an object of type PermissionResponse
.
Types
Type: AppleMapsMarker
extended by:
Property | Type | Description |
---|---|---|
backgroundColor(optional) | string | The background color of the annotation. |
icon(optional) | SharedRefType<'image'> | The custom icon to display in the annotation. |
text(optional) | string | The text to display in the annotation. |
textColor(optional) | string | The text color of the annotation. |
Property | Type | Description |
---|---|---|
coordinates(optional) | Coordinates | The coordinates of the marker. |
id(optional) | string | The unique identifier for the marker. This can be used to e.g. identify the clicked marker in the |
systemImage(optional) | string | The SF Symbol to display for the marker. |
tintColor(optional) | string | The tint color of the marker. |
title(optional) | string | The title of the marker, displayed in the callout when the marker is clicked. |
Property | Type | Description |
---|---|---|
color(optional) | ProcessedColorValue | string | The color of the polyline. |
contourStyle(optional) | AppleMapsContourStyle | The style of the polyline. |
coordinates | Coordinates[] | The coordinates of the polyline. |
id(optional) | string | The unique identifier for the polyline. This can be used to e.g. identify the clicked polyline in the |
width(optional) | number | The width of the polyline. |
Property | Type | Description |
---|---|---|
isTrafficEnabled(optional) | boolean | Whether the traffic layer is enabled on the map. |
mapType(optional) | AppleMapsMapType | Defines which map type should be used. |
polylineTapThreshold(optional) | number | The maximum distance in meters from a tap of a polyline for it to be considered a hit.
If the distance is greater than the threshold, the polyline is not considered a hit.
If a hit occurs, the |
selectionEnabled(optional) | boolean | If true, the user can select a location on the map to get more information. |
Property | Type | Description |
---|---|---|
compassEnabled(optional) | boolean | Whether the compass is enabled on the map. If enabled, the compass is only visible when the map is rotated. |
myLocationButtonEnabled(optional) | boolean | Whether the my location button is visible. |
scaleBarEnabled(optional) | boolean | Whether the scale bar is displayed when zooming. |
togglePitchEnabled(optional) | boolean | Whether the user is allowed to change the pitch type. |
Property | Type | Description |
---|---|---|
setCameraPosition | (config: CameraPosition) => void | Update camera position. Animation duration is not supported on iOS. config: CameraPosition New camera postion. |
Property | Type | Description |
---|---|---|
coordinates(optional) | Coordinates | The middle point of the camera. |
zoom(optional) | number | The zoom level of the camera. For some view sizes, lower zoom levels might not be available. |
Property | Type | Description |
---|---|---|
latitude(optional) | number | The latitude of the coordinate. |
longitude(optional) | number | The longitude of the coordinate. |
Property | Type | Description |
---|---|---|
coordinates(optional) | Coordinates | The coordinates of the marker. |
draggable(optional) | boolean | Whether the marker is draggable. |
icon(optional) | SharedRefType<'image'> | The custom icon to display for the marker. |
id(optional) | string | The unique identifier for the marker. This can be used to e.g. identify the clicked marker in the |
showCallout(optional) | boolean | Whether the callout should be shown when the marker is clicked. |
snippet(optional) | string | The snippet of the marker, Displayed in the callout when the marker is clicked. |
title(optional) | string | The title of the marker, displayed in the callout when the marker is clicked. |
Property | Type | Description |
---|---|---|
color(optional) | ProcessedColorValue | string | The color of the polyline. |
coordinates | Coordinates[] | The coordinates of the polyline. |
geodesic(optional) | boolean | Whether the polyline is geodesic. |
id(optional) | string | The unique identifier for the polyline. This can be used to e.g. identify the clicked polyline in the |
width(optional) | number | The width of the polyline. |
Property | Type | Description |
---|---|---|
isBuildingEnabled(optional) | boolean | Whether the building layer is enabled on the map. |
isIndoorEnabled(optional) | boolean | Whether the indoor layer is enabled on the map. |
isMyLocationEnabled(optional) | boolean | Whether finding the user's location is enabled on the map. |
isTrafficEnabled(optional) | boolean | Whether the traffic layer is enabled on the map. |
mapType(optional) | GoogleMapsMapType | Defines which map type should be used. |
maxZoomPreference(optional) | number | The maximum zoom level for the map. |
minZoomPreference(optional) | number | The minimum zoom level for the map. |
selectionEnabled(optional) | boolean | If true, the user can select a location on the map to get more information. |
Property | Type | Description |
---|---|---|
compassEnabled(optional) | boolean | Whether the compass is enabled on the map. If enabled, the compass is only visible when the map is rotated. |
indoorLevelPickerEnabled(optional) | boolean | Whether the indoor level picker is enabled . |
mapToolbarEnabled(optional) | boolean | Whether the map toolbar is visible. |
myLocationButtonEnabled(optional) | boolean | Whether the my location button is visible. |
rotationGesturesEnabled(optional) | boolean | Whether rotate gestures are enabled. |
scaleBarEnabled(optional) | boolean | Whether the scale bar is displayed when zooming. |
scrollGesturesEnabled(optional) | boolean | Whether the scroll gestures are enabled. |
scrollGesturesEnabledDuringRotateOrZoom(optional) | boolean | Whether the scroll gestures are enabled during rotation or zoom. |
tiltGesturesEnabled(optional) | boolean | Whether the tilt gestures are enabled. |
togglePitchEnabled(optional) | boolean | Whether the user is allowed to change the pitch type. |
zoomControlsEnabled(optional) | boolean | Whether the zoom controls are visible. |
zoomGesturesEnabled(optional) | boolean | Whether the zoom gestures are enabled. |
Property | Type | Description |
---|---|---|
coordinates | Coordinates | User location coordinates. |
followUserLocation | boolean | Should the camera follow the users' location. |
Property | Type | Description |
---|---|---|
setCameraPosition | (config: SetCameraPositionConfig) => void | Update camera position. config: SetCameraPositionConfig New camera position config. |
Type: CameraPosition
extended by:
Property | Type | Description |
---|---|---|
duration(optional) | number | The duration of the animation in milliseconds. |
Property | Type | Description |
---|---|---|
bearing(optional) | number | - |
coordinates | Coordinates | - |
tilt(optional) | number | - |
zoom(optional) | number | - |
Enums
The style of the polyline.
The type of map to display.
AppleMapsMapType.HYBRID = "HYBRID"
A satellite image of the area with road and road name layers on top.
The type of map to display.
GoogleMapsMapType.HYBRID = "HYBRID"
Satellite imagery with roads and points of interest overlayed.
Permissions
Android
To show the user's location on the map, the expo-maps
library requires the following permissions:
ACCESS_COARSE_LOCATION
: for approximate device locationACCESS_FINE_LOCATION
: for precise device location
Android Permission | Description |
---|---|
Allows an app to access approximate location.
| |
Allows an app to access precise location.
| |
Allows a regular application to use Service.startForeground.
| |
Allows a regular application to use Service.startForeground with the type "location".
| |
Allows an app to access location in the background.
|
iOS
The following usage description keys are used by this library: