处理平台差异
在本教程中,了解在创建通用应用时如何处理原生和 Web 之间的平台差异。
Android、iOS 和 Web 具有不同的功能。在我们的例子中,Android 和 iOS 都可以使用 react-native-view-shot
库捕获屏幕截图。但是,Web 浏览器不能。
¥Android, iOS, and the web have different capabilities. In our case, both Android and iOS can capture a screenshot with the react-native-view-shot
library. However, web browsers cannot.
在本章中,我们将学习如何处理 Web 浏览器的屏幕截图,以便我们的应用在所有平台上具有相同的功能。
¥In this chapter, we'll learn how to handle capturing screenshots for web browsers so our app has the same functionality on all platforms.
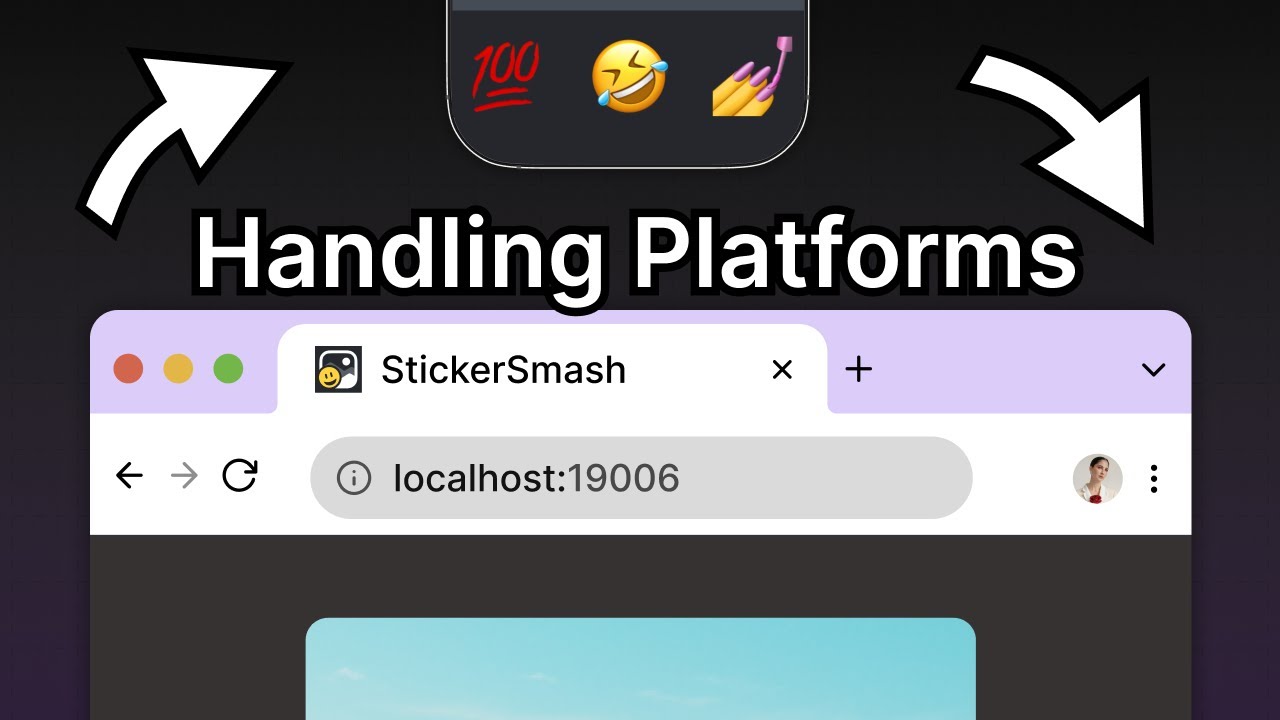
1
Install and import dom-to-image
To capture a screenshot on the web and save it as an image, we'll use a third-party library called dom-to-image
. It takes a screenshot of any DOM node and turns it into a vector (SVG) or raster (PNG or JPEG) image.
Stop the development server and run the following command to install the library:
!!!IG2!!!
After installing it, make sure to restart the development server and press w in the terminal.
2
Add platform-specific code
Using Platform
module from React Native, we can implement platform-specific behavior. Inside app/(tabs)/index.tsx:
- Import the
Platform
module fromreact-native
. - Import the
domtoimage
library fromdom-to-image
. - Update the
onSaveImageAsync()
function to check whether the current platform is'web'
with thePlatform.OS
property. If it is'web'
, we'll use thedomtoimage.toJpeg()
method to convert and capture the current<View>
as a JPEG image. Otherwise, we'll keep using the same logic added for native platforms.
!!!IG3!!!
!!!IG0!!!
Fix dom-to-image
TypeScript module error
We need to add a type definition after importing the domtoimage
library since we're using TypeScript. We can do this by creating a file types.d.ts in the root of our project directory and adding the declaration statement:
!!!IG1!!!
On running the app in a web browser, we can now save a screenshot:
Summary
Chapter 8: Handle platform differences
The app does everything we set out for it to do, so it's time to shift our focus toward the purely aesthetic..
In the next chapter, we will customize the app's status bar, splash screen, and app icon.